|
<binding.http> Introduction
The Tuscany Java SCA runtime supports Hyper Text Transfer Protocol (HTTP) using the <binding.http> extension. Tuscany can communicate with services that provide or consume business data objects via the well known actions of HTTP, for example POST, GET, PUT, and DELETE. In HTTP interactions between a client and a server takes place as a series of requests and responses. Information is comunicated by reads and rights over server socket ports. HTTP actions, also known as verbs, are communicated between a client and a server in the requests and responses. Each request and response consists of a header and a body. Typically the header contains the request action name, a URI indicating the location of object of the object of the action, and a number property name and value pairs containing other meta information about the transaction (e.g. body length, modification dates, MIME type, etc.). The body contains the subject of the action, whether it be a text or binary encoding of the data, an error message, or a serialized object.
More information on the HTTP protocol is located at:
Some of the advanced function described here is included in the Tuscany 1.3.2 and 1.4 releases. The complete timeline of available and future plans is given in the Tuscany Web 2.0 Roadmap. Users should also be aware of the Atom binding and RSS binding which sit on top of the HTTP binding, but have additional features and data types associated with the actions.
A Tuscany protocol binding such as the HTTP binding, is a way to fit a common protocol into the Tuscany abstraction and way of doing things. Each binding identifies itself to the Tuscany runtime and states under what conditions it is available. The Tuscany runtime uses the binding to translate invocations and data from the Tuscany world into the world of the protocol and back. So in the case of the HTTP binding, Tuscany uses the HTTP request and response mechanism to share business data between service components.
Using the Tuscany HTTP binding
The primary use of the HTTP binding is to share resources and services over HTTP over the web in a distributed fashion. Resources are items that have a resource implementation such as web content. Services are items that have data types and a defined business interfaces such as shared collections. Examples of shared collections includes shopping carts, telephone directories, insurance forms, and blog sites. These collections of items can be added, retrieved, updated, and deleted using the 4 basic actions of the HTTP protocol:
- POST (create or add)
- GET (retreive or query)
- PUT (update)
- DELETE (destroy or remove
The simplest way to use the HTTP binding is to declare a resource that can be shared over the web via HTTP and provide an HTTP address where one can access the resource. This resource is declared in an SCA composite file which describes the SCA domain.
<component name="ResourceServiceComponent">
<tuscany:implementation.resource location="content"/>
<service name="Resource">
<tuscany:binding.http uri="http:/>
</service>
</component>
No further implementation is needed with a resource. It is served on the web like any other static web content.
The HTTP binding can also declare a business service that can be shared over the web and provide an HTTP address where one can access the service. This resource is declared in an SCA composite file which describes the SCA domain.
<component name="HTTPBindingComponent">
<implementation.java class="org.apache.tuscany.sca.binding.http.TestBindingImpl"/>
<service name="TestBindingImpl">
<tuscany:binding.http uri="http:/>
</service>
</component>
Example HTTP Servlet and Service Implementations
A service that uses the HTTP binding usually implements the javax.servlet.Servlet interface. This interface declares the basic access methods mentioned in the J2EE specification: init, destroy, service, getServletInfo, etc. The Tuscany runtime ensures that the proper method is invoked whenever a service does one of the HTTP actions. For example here is a TestService implemented in the package org.apache.tuscany.sca.binding.http;
@Service(Servlet.class)
public class TestServiceImpl implements Servlet {
public void init(ServletConfig config) throws ServletException {
}
public void service(ServletRequest request, ServletResponse response) throws ServletException, IOException {
response.getOutputStream().print("<html><body><p>Hello from Tuscany HTTP service</body></html>");
}
}
Another way of implementing an HTTP service is to use a collection interface that matches the actions of the HTTP protocol. In this case, the methods must be named post, get, put, and delete. Tuscany ensures that the proper method is invoked via the request and response protocol of HTTP:
public class TestGetImpl {
public InputStream get(String id) {
return new ByteArrayInputStream(("<html><body><p>This is the service GET method, item=" + id + "</body></html>").getBytes());
}
}
So using the common verbs of HTTP and Java object serialization, one can implement services and run them anywhere the HTTP protocol is implemented. The service developer or implementer simply creates methods for post, get, put, and delete, and a business collection such as a shopping cart, telephone directory, insurance form, or blog sites can be created. See the Tuscany module binding-http-runtime for complete examples.
Unlike the Atom or RSS bindings, which have defined data types which encapsulate the business objects, the HTTP binding uses Java object serialization for passing business object data back and forth. Thus it is up to the developer or implementer to deserialize the data and reconstitute a business object.
Advanced Features of the Tuscany HTTP Binding
HTTP Conditional Actions and Caching using ETags and Last-Modified
The HTTP specification provides a set of methods for HTTP clients and servers to interact. These methods form the foundation of the World Wide Web. Tuscany implements many of these methods a binding interface to a collection. The main methods are:
- GET - retrieves an item from a collection
- POST - creates or adds an item to a collection
- PUT - updates or replaces an item in a collection
- DELETE - removes an item in a a collection
The HTTP specification (HTTP 1.1 Chapter 13 - Caching) also provides a mechanism by which these methods may be executed conditionally. To perform conditional methods, an HTTP client puts 3 items in the HTTP request header:
- ETag - entity tag, a unique identifier to an item in a collection. Normally created and returned by the server when creating (POST) a new item.
- LastModified - an updated field. Normally a string containing a date and time of the last modification of the item.
- Predicate - a logical test (e.g. IfModified, IfUnmodified) to use with the ETag and LastModified to determine whether to act.
The complete list of predicates is given in the HTTP specification.
The most common use of conditional methods is to prevent two requests to the server instead of one conditional request. For example, a common scenario is to check if an item has been modified, if not changed update it with a new version, if changed do not update it. With a conditional PUT method (using the IfUnmodifed predicate and a LastModified date), this can be done in one action. Another common use is to prevent multiple GETs of an item to ensure we have a valid copy. Rather than doing a second request of a large item, one can do a conditional GET request (using an IfModified predicate and a LastModified date), and avoid the second request if our object is still valid. The server responds with either a normal response body, or status code 304 (Not Modified), or status code 412 (precondition failed).
Tuscany implements the logic to move these caching items to and from the HTTP request and response headers, and deliver them to the collection implementation. The items are delivered to a user implementation via a serializable object called CacheContext. This object contains the value of the ETag, the LastModified value, and any predicates. It is up to the implementer of a collection to provide the correct server logic to act on these predicates. The CacheContext class is found in found in the Tuscany module package org.apache.tuscany.sca.binding.http.
To implement conditional actions on your service, the developer or implementer implements any of the condional HTTP actions: conditionalPost, conditionalGet, conditionalPut, or conditionalDelete. Tuscany automatically routes a request with proper request header fields (ETag, LastModified, and predicates) to the proper collection method.
For example, the TestBindingCacheImpl class in package org.apache.tuscany.sca.binding.http implements a server collection which pays attention to conditional methods. Notice that this collection implements conditionalGet, conditionalPut, conditionalPost, and conditionalDelete methods as well as get, put, post, delete. The server collection can look at the CacheContext obect to determine whether an item or a status code should be returned. In this example code, the conditionalGet checked the If-Modified predicate and determines whether the item is not modified and if so, throws a NotModifiedException.
public InputStream conditionalGet(String id, HTTPCacheContext cacheContext)
throws NotModifiedException, PreconditionFailedException {
if (cacheContext != null) {
if (cacheContext.ifModifiedSince) {
if ((id.equals("1"))
&& (0 > cacheContext.lastModifiedDate
.compareTo(new Date())))
throw new NotModifiedException("item 1 was modified on "
+ new Date());
}
...
...
For a full example of all conditional methods and many combinations of predicates, one can look to the module http-binding unit tests to understand how these conditional request work. The HTTPBindingCacheTestCase contains 36 tests of all 4 HTTP conditonal methods. Various predicates are tested with various ETags and LastModified fields. The fields are tested in both the positive and negative cases.
Here is a complete list of the tests:
- testGet() - tests normal GET method of collection, expects item
- testConditionalGetIfModifiedNegative() - tests not modified GET, expects item
- testConditionalGetIfModifiedPositive() - tests modified GET, expect code 304
- testConditionalGetIfUnmodifiedNegative() - tests unmodifed GET, expects item
- testConditionalGetIfUnmodifiedPositive() - tests modified GET, expects code 412
- testConditionalGetIfMatchNegative() - tests matching GET, expects code 412
- testConditionalGetIfMatchPositive() - tests matching GET, expects item
- testConditionalGetIfNoneMatchNegative - tests unmatching GET, expects item
- testConditionalGetIfNoneMatchPositive() - tests unmatching GET, expects code 412
Similarly, there are 9 tests each for DELETE, POST, and PUT, making a total of 36 test cases.
Differences Between HTTP Binding Caching and Atom Binding Caching in Tuscany
A similar sort of conditional posting is implemented in the Atom Binding. The difference is that the HTTP binding requires an implementer to provide conditional methods. The Atom binding caching uses the Abdera Atom model objects to automatically derive an ETag and a LastModified tags. In the Atom model, the Entry or Feed ID is used to make an item ETag field. The Atom Entry or Feed updated field is used to make an item LastModified field.
Use the HTTP binding caching when you are not using Atom Entries and Feeds as your business objects. However, the more flexible HTTP binding caching requires you to implement special conditional methods. Use the Atom binding when you can model your business data in terms of Atom Entries and Feeds. The more constrictive Atom binding caching will derive caching tags from your data model fields.
Security Policy support in HTTP and Web 2.0 Bindings
 | work in progress |
Scenarios
- A Web 2.0 application requires that a user get authenticated before it can access the application.
- A Web 2.0 application requires that all communication between client/server be done using SSL.
- A given service, exposed using a web 2.0 binding requires user authentication.
- A given operation, exposed using a web 2.0 binding requires user authentication.
Policy Interceptor
The design approach that is being considered is to inject policy security interceptors, that would properly validate and enforce the security intents.
The authentication will be done using JAAS modules for authentication, and initially we would support authenticating to a list of username/password supplied by the application or using an LDAP.
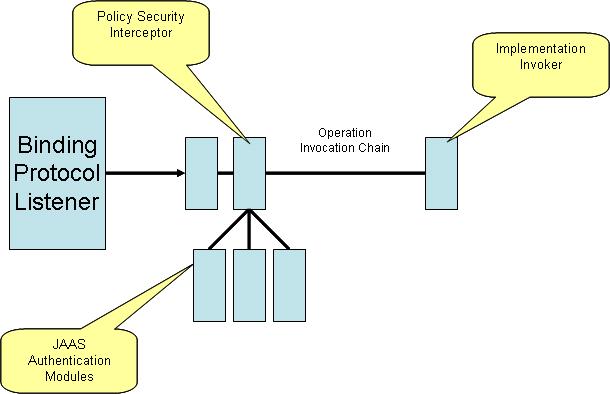
|